By someone who has broken more agents than they care to admit—and learned a thing or two in the process.
1. Introduction: How to Make an AI Agent
Last winter, I read an article “how to make an AI agent” and wrote a tiny Python script for it that watched my inbox and shifted every flight confirmation into a neatly labeled folder. If you’re curious how to make an AI agent, consider that this one‑evening hack—just forty‑seven lines of code, half of which were print statements—already felt alive the next morning when I imagined it hunting for cheaper seats, rebooking flights, and texting me refunds. That thought sparked the vision of miniature software servants that see, remember, decide, and act without my constant babysitting.
That, at heart, is the promise of an AI agent: squeeze just enough intelligence into a loop of perception → reasoning → action so the code starts shaping the world on its own. When that loop tightens and scales through AI agent orchestration, you unlock more than convenience—you change how work itself is carved up between silicon and neurons.
The rest of this piece is a road map for How to Make an AI Agent—equal parts conceptual compass and wrench‑ready tutorial. I’ll assume you can write a function and read a traceback; everything else we’ll build from scratch. For more AI insights, visit our Binary Verse AI blog.
Table of Contents
Summary of “How to Make an AI Agent” for people in a hurry
Introduction: Why We Bother
- A simple Python script automated sorting flight confirmations.
- Vision emerged of agents rebooking flights and texting refunds.
- Illustrates the perception → reasoning → action loop.
- Miniature software servants can operate without babysitting.
- Intelligent loops reshape division of labor between code and humans.
- AI agents offer more than convenience—they transform workflows.
- This guide blends conceptual overview with hands‑on tutorial.
Anatomy of an Agent
- Perception turns raw inputs (APIs, pixels, chat) into tokens.
- Short‑term memory stores context for a single turn.
- Long‑term memory persists facts via embeddings and vector stores.
- Planning cortex decomposes goals into atomic tasks.
- Actuators execute actions via functions, endpoints, or hardware.
- Removing any organ breaks the illusion of autonomy.
- All five organs enable adaptive, autonomous behavior.
Solo vs. Swarm
- Solo agents are focused but can fail if left unmanaged.
- Swarms enable parallelism for faster completion.
- Fault tolerance: one agent’s crash doesn’t halt the swarm.
- Emergent behavior arises from coordinated interactions.
- Orchestration overhead adds protocol and state complexity.
- Swarm debugging is harder due to asynchronous logs.
- Start solo, add swarm when tasks naturally decompose.
Choosing Your Toolkit
- Dify: drag‑drop canvas for quick chatbot proofs.
- LangChain: modular “Load”, “Split”, “Embed” verbs.
- Semantic Kernel: C# integration with durable state machines.
- AutoGen: async flows and human‑in‑loop conversation patterns.
- SmolAgents: minimal 1k LOC sandboxed Python agents.
- All tools are open source and update weekly.
- Choose tools that shorten path from idea to demo.
Step by Step: Building in Python
- Install prerequisites:
pip install requests python-dotenv
. - Load API key via
dotenv
or environment variable. - Maintain a sliding memory buffer of recent inputs.
- Define a calculator tool for basic math evaluation.
- Wrap the Gemini API call with JSON payload and headers.
- Orchestrate loop: route math vs. LLM calls and print results.
- Demonstrates the full perceive → plan → act → remember cycle.
Retrieval‑Augmented Generation (RAG)
- Chunk size (~400 words) impacts retrieval quality.
- Hybrid search blends dense embeddings with keyword filters.
- Append source URLs to surface provenance.
- Proper chunking avoids duplicates and context overrun.
- TF‑IDF can rescue cosine‑similarity misses.
- RAG grounds generations in relevant external data.
- Transparency builds user trust over hidden LLM outputs.
NLP Edge Cases
- Anaphora can cause ambiguous references (“it”, “there”).
- Sarcasm misleads models without guardrails.
- Reflexive clarifications improve intent accuracy.
- Lightweight regex filters catch confusing phrases.
- Good prompts often suffice without complex NLU heads.
- Domain‑specific NLU models are overkill early on.
- Escalate to complex solutions only when metrics plateau.
Explainability, Ethics & Security
- Log internal chain of thought for auditability.
- Redact sensitive reasoning before user display.
- Add moderation layers on inputs and outputs.
- Sandbox code execution to prevent misuse.
- Sign and rotate API keys regularly.
- Rate‑limit requests to thwart abuse and injection.
- Guard against prompt injection with strict validation.
Orchestrating a Crew
- Split pipeline into specialized roles (researcher, writer, etc.).
- Use JSON or similar shared formats for communication.
- Introduce a conductor component for task routing.
- Begin with synchronous flows before parallelizing.
- Define clear handoff protocols between agents.
- Plan shared state and security rules carefully.
- Enables end‑to‑end automated content pipelines.
A Note on Imperfection
- Production agents often launch with bugs and loops.
- Observability via OpenTelemetry or LangSmith is vital.
- Track metrics on tool latency and error rates.
- Dashboards reveal hidden bottlenecks.
- Reliability grows from visibility, not heroics.
- Plan for silent failures and graceful recoveries.
- Iterate instrumentation alongside new features.
Conclusion: Looking Forward
- LLMs will get cheaper and context windows longer.
- “AI agent” will soon be as common as “web app.”
- Task decomposition and guardrails remain critical skills.
- Governance and ethics outlive current model capabilities.
- Master fundamentals now for tomorrow’s tooling drops.
- Small autonomies free human attention for higher‑order work.
- Your turn: build, break, and learn from your own agents.
2. Anatomy of How to Make an AI Agent (In Plain English)
Picture an agent as a five‑organ organism, a helpful analogy from AWS – What are AI Agents?
- Eyes & Ears (Perception): Raw inputs—API payloads, webcam pixels, user chat—get turned into structured tokens a model can chew on.
- Short Term Memory: A scratchpad that survives a single conversation turn or decision step. Lose it and you’ll repeat yourself like a goldfish.
- Long Term Memory: Facts, rules, or entire document stores that outlive the process—think embeddings and vector databases.
- Planning Cortex: Breaks a vague goal (“book the cheapest refundable flight to Seoul”) into atomic commands: search, compare, reserve, confirm, notify.
- Actuators (Tools): Python functions, HTTP endpoints, robotic arms—whatever actually pushes on the outside world.
Strip any organ away and the illusion of autonomy cracks. With all five clicking, you get software that feels alive: it notices, deliberates, remembers, and adjusts.
3. Solo vs. Swarm
A single‑agent system is a friendly golden retriever: eager, focused, occasionally eats your homework if you leave it alone too long. A multi‑agent swarm is more like an ant colony—tiny specialists exchanging signals to move mountains of sand one grain at a time.
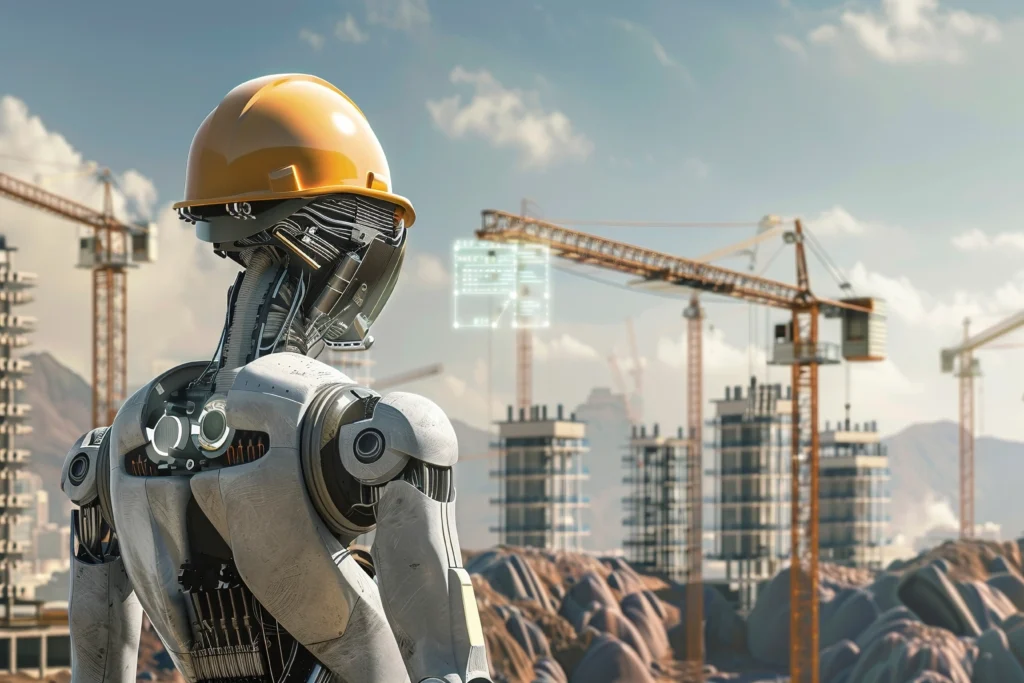
Swarm perks:
- Parallelism – Ten narrow agents beat one bloated one on wall‑clock time.
- Fault tolerance – If the “Link Checker” crashes, the “Answerer” still operates.
- Emergent behavior – Coordination algorithms (stigmergy, auctions, role playing) surface solutions no single agent could design.
Drawbacks:
- Orchestration overhead – Agreeing on protocols, shared state, security rules.
- Debugging nightmares – Logs multiply; causality hides behind asynchronous messages.
My rule of thumb: start solo, then add friends only when the task graph naturally decomposes.
4. Choosing Your Toolkit for How to Make an AI Agent (A Biased Cheat Sheet)
Need | Sweet Spot Framework | Why I Reach for It |
Quick proof‑of‑concept chatbot | Dify | Drag‑drop canvas; free GPU tier; JSON logs that make sense. |
Pythonic Swiss Army knife | LangChain | Modular verbs (“Load”, “Split”, “Embed”, “Search”) you can rearrange like Lego. |
Enterprise workflow, .NET shop | Semantic Kernel | Plays nice with C#; durable state machines baked in. |
Research on agent cooperation | AutoGen | Conversation patterns, easy human‑in‑the‑loop, async by default. |
Minimalist hacking, code‑writing agents | SmolAgents | ~1k LOC, sandboxes generated Python, nothing else. |
All are open source and improve weekly. Pick whatever shortens the gap between idea and first demo—migration later is rarely traumatic.
5. Step by Step: How to Make an AI Agent in Python
Below is the complete code snippet:
# pip install requests python-dotenv import os import requests from dotenv import load_dotenv # ─── Load your Gemini key ───────────────────────────────────────────────────── load_dotenv() # comment out if you don’t use a .env file API_KEY = "Paste your API Key Here" # Gemini endpoint API_URL = f"https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key={API_KEY}" # ─── Minimal in‑memory chat history ──────────────────────────────────────────── chat_history = [] # ─── Calculator “tool” ───────────────────────────────────────────────────────── def calculator_tool(expr: str) -> str: """Evaluate a simple arithmetic expression.""" try: # WARNING: eval can be dangerous; only use for trusted input or sandbox it. return str(eval(expr, {}, {})) except Exception as e: return f"Calc error: {e}" # ─── Core Gemini call ────────────────────────────────────────────────────────── def call_gemini(prompt: str) -> str: """Send the prompt to Gemini and return its reply text.""" headers = {"Content-Type": "application/json"} payload = { "contents": [{"role": "user", "parts": [{"text": prompt}]}], "generationConfig": { "temperature": 0.2, "topK": 1, "topP": 1.0, "maxOutputTokens": 2048, }, } params = {"key": API_KEY} resp = requests.post(API_URL, headers=headers, params=params, json=payload) resp.raise_for_status() data = resp.json().get("candidates", [{}])[0] return data.get("content", {}).get("parts", [{}])[0].get("text", "") # ─── Agent loop ──────────────────────────────────────────────────────────────── def run_agent(): print("Simple Gemini‑powered Agent (type 'quit' to exit)\n") while True: user_input = input(">> ").strip() if user_input.lower() in {"quit", "exit"}: print("Goodbye!") break # Very basic math detection: math_chars = set("0123456789+-*/(). ") if set(user_input) <= math_chars and any(c in user_input for c in "+-*/"): # Route to calculator result = calculator_tool(user_input) else: # Maintain a sliding window of last 5 turns chat_history.append(user_input) if len(chat_history) > 5: chat_history.pop(0) # Build prompt from history prompt = "\n".join(f"User: {q}" for q in chat_history) result = call_gemini(prompt) print(result, "\n") if __name__ == "__main__": run_agent()
Step‑by‑Step Guide
The following script qualifies as a basic AI agent rather than just a chatbot, because it combines perception, memory, reasoning/planning, action, and learning.
Install prerequisites:
nginxCopyEditpip install requests python-dotenv
Load your API key to start How to Make an AI Agent:
pythonCopyEditfrom dotenv import load_dotenv
load_dotenv()
API_KEY = os.getenv("GEMINI_API_KEY") or "your-key-here"
API_URL = "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent"
Maintain a simple memory buffer for How to Make an AI Agent:
pythonCopyEditchat_history = []
Define the calculator tool in your How to Make an AI Agent implementation:
pythonCopyEditdef calculator_tool(expr: str) -> str:
try:
return str(eval(expr, {}, {}))
except Exception as e:
return f"Calc error: {e}"
Wrap the Gemini API call to complete How to Make an AI Agent:
pythonCopyEditdef call_gemini(prompt: str) -> str:
headers = {"Content-Type": "application/json"}
payload = {
"contents": [{"role":"user","parts":[{"text":prompt}]}],
"generationConfig": {
"temperature": 0.2,
"topK": 1,
"topP": 1.0,
"maxOutputTokens": 2048
}
}
resp = requests.post(
API_URL,
headers=headers,
params={"key": API_KEY},
json=payload
)
resp.raise_for_status()
data = resp.json().get("candidates", [{}])[0]
return data.get("content", {}).get("parts", [{}])[0].get("text", "")
Orchestrate the main loop to finalize How to Make an AI Agent:
pythonCopyEditdef run_agent():
print("Agent (type 'quit' to exit)")
while True:
user_input = input(">> ").strip()
if user_input.lower() in {"quit", "exit"}:
break
# If purely arithmetic
if set(user_input) <= set("0123456789+-*/(). ") and any(c in user_input for c in "+-*/"):
result = calculator_tool(user_input)
else:
chat_history.append(user_input)
if len(chat_history) > 5:
chat_history.pop(0)
prompt = "\n".join(f"User: {q}" for q in chat_history)
result = call_gemini(prompt)
print(result, "\n")
Why We Call It an Agent in How to Make an AI Agent:
- It demonstrates How to Make an AI Agent autonomously chooses which tool to invoke.
- It preserves conversational context, showing How to Make an AI Agent is context‑aware.
- It integrates multiple capabilities (math, LLM).
- It follows the perceive → plan → act → remember loop.
Adding More Tools to How to Make an AI Agent:
- Utilities & Math for How to Make an AI Agent; Math
- Calculator
Evaluate arbitrary arithmetic expressions (already in use). - Unit Converter
Convert units (e.g.convert("5 miles to km")
→8.047
). - Date/Time Tool
Parse or compute dates (e.g. “add 7 days to 2025‑04‑22”). - Random Generator
Roll dice, pick a random element, generate passwords. - Regex Extractor
Extract or validate patterns (emails, phone numbers) via Python’sre
.
- Calculator
- Web & Data Retrieval in How to Make an AI Agent
- WebSearch
Hit a search‑engine API and return top results. - HTTP Client
Fetch URLs and return HTML/text or JSON. - RSS Reader
Pull headlines from any RSS/Atom feed. - Stock Quotes
Use a finance API to get real‑time prices. - Cryptocurrency Ticker
Query CoinGecko or CoinMarketCap for crypto prices.
- WebSearch
- File & Document Processing
- Text File Reader
Load.txt
or Markdown files and feed content to Gemini. - PDF Summarizer
Extract text from PDFs (via PyPDF2 or pdfplumber) and summarize. - Spreadsheet Query
Read.csv
/.xlsx
(with pandas) and answer questions (“average sales Q1”). - Image OCR
Use Tesseract or a cloud OCR API to extract text from images. - Document Translator
Auto‑translate entire documents via Google/Azure Translate.
- Text File Reader
- Productivity & Scheduling
- Calendar Manager
Create, query, or delete events in Google Calendar or Outlook. - Timer/Reminder
Schedule an alert or send an email/SMS at a future time. - Email Sender
Send templated emails via SMTP or an API (SendGrid, SES). - To‑Do List
Append tasks to a text file, Trello board, or Notion database. - Meeting Link Generator
Programmatically create Zoom/Teams/Google Meet links.
- Calendar Manager
- Communication & Social
- Slack Bot
Post messages to a Slack channel or read channel history. - Twitter Client
Fetch or post tweets via the Twitter/X API. - SMS Gateway
Send/receive texts via Twilio or similar services. - Discord Notifier
Send alerts to a Discord webhook. - Telegram Bot
Interact with users, fetch updates, or push notifications.
- Slack Bot
- Data & Knowledge → RAG
- Vector Retriever
Connect to a FAISS or Pinecone index for embedding search. - SQL Query Runner
Execute SQL against Postgres/MySQL and return tabular results. - Knowledge‑Base Lookup
Fetch entries from a Wiki, SharePoint, or Airtable. - Web‑Scraper
Crawl sites for fields (e.g. product prices) using BeautifulSoup. - API Orchestrator
Fan‑out calls to multiple APIs (e.g. price compare across e‑commerce sites).
- Vector Retriever
- AI & ML Helpers
- Sentiment Analyzer
Score sentiment via an open‑source model or API. - Entity Extractor
Identify names, dates, locations via spaCy or an LLM prompt. - Summarizer
Condense long texts to bullet points. - Classifier
Label text by category (spam/ham, topic tags) using a pretrained model. - Image Generator
Hook into Stable Diffusion or DALL·E to produce images from text prompts.
- Sentiment Analyzer
- DevOps & Monitoring
- Kubernetes Controller
Scale deployments or fetch pod status via kubernetes-client. - Docker Manager
Spin up, stop, or inspect containers using Docker SDK for Python. - CI Trigger
Kick off a GitHub Actions or Jenkins job via its REST API. - Log Fetcher
Pull the last N lines from CloudWatch, ELK, or local log files. - Health‑Check
Ping URLs or TCP ports and report failures.
- Kubernetes Controller
How to Integrate a New Tool
- Write a Python function that takes an input (string or otherwise) and returns a string result.
- Decide where in your
run_agent()
loop it should fire—e.g. by keyword matching, regex, or a tiny intent model. - Route that input into your function, capture its output, and
print(...)
it instead of calling Gemini.
6. Retrieval Augmented Generation Done Right
RAG is the duct tape that holds agent facts together. Three tips you won’t regret:
- Chunk size is destiny. If your splitter creates 3‑token blobs, your retriever drowns in near‑duplicates; 3,000‑token slabs blow the context budget. I default to ~400 words, then tune.
- Use hybrid search. Combine dense embeddings with a cheap keyword filter. Surprising how often TF–IDF rescues a cosine‑similarity miss.
- Surface provenance. Append “Source: {url}” after each paragraph. Users forgive occasional errors; they loathe mystery meat.
7. Talk Like a Human: NLP Edge Cases
LLMs are brilliant until they face anaphora (“Move that file to the bucket where we saved it yesterday”) or sarcasm (“Oh great, another billing error”). Two battle‑tested tricks:
- Reflexive clarifications. When intent confidence drops, the agent should ask—“By ‘it’, do you mean the invoice PDF or the spreadsheet?”
- Lightweight rules. A three‑line regex catching “thanks but” or “lol sure” before feeding user text into the model avoids surprisingly many misunderstandings.
Training a domain‑specific NLU headliner is tempting but often overkill. Start with good prompts and guardrails; escalate only when metrics stall.
8. Safety Valve: Explainability, Ethics, Security
- Explainability – Log chain of thought internally, redact it for users. Snapshot every tool call and response to troubleshoot OOMs or late‑night failures.
- Ethics – Install a moderation layer on both inputs and outputs to prevent auto‑sending harmful content.
- Security – Sandbox code execution, sign API requests, rotate keys, rate‑limit aggressively. Prompt injection isn’t theoretical; attackers already chain LLM tasks to exfiltrate secrets.
9. Stepping Beyond One Brain: Orchestrating a Crew
Imagine a content pipeline:
- Researcher gathers references
- Writer drafts 1,000 words
- Editor polishes tone and fact‑checks
- SEO Bot injects metadata
- Scheduler publishes at low‑traffic hours
With a multi‑agent orchestration (agentic AI), that’s five specialized agents passing a baton—each with its own tools and constraints. The trick is designing a shared language (usually JSON messages) and a conductor that decides who speaks next. Keep first iterations synchronous—parallelism can wait.
10. A Note on Imperfection
Every production agent I’ve shipped launched with a glaring flaw: infinite loops, silent 500s, comedic misunderstandings (“Cancel my mom” instead of “Cancel with my mom”). The cure isn’t heroics; it’s observability: OpenTelemetry traces, LangSmith dashboards, metrics on tool latency. When visibility improves, reliability follows.
11. Conclusion: Looking Forward
LLMs will get cheaper, context windows will stretch, and eventually “AI agent” will sound as quaint as “web app.” The hard parts—task decomposition, guardrails, governance—will outlive today’s models. Master those fundamentals now and tomorrow’s tooling drop is pure upside.
I still haven’t built the self‑rebooking flight agent. But last week my inbox script gained a new trick: it texts me “Prices dropped by $87—want me to reissue?” I reply “Y,” and it does the rest while I sip coffee. A modest victory, sure, yet it reminds us why we keep iterating: each small autonomy frees a slice of human attention for things only humans can do.
Comments, pull requests, or war stories welcome via contact us. Source code lives on GitHub; links in the footer.
Credit to Freepik